(python)chatGPT로 파일 읽고 쓰기 코딩 연습
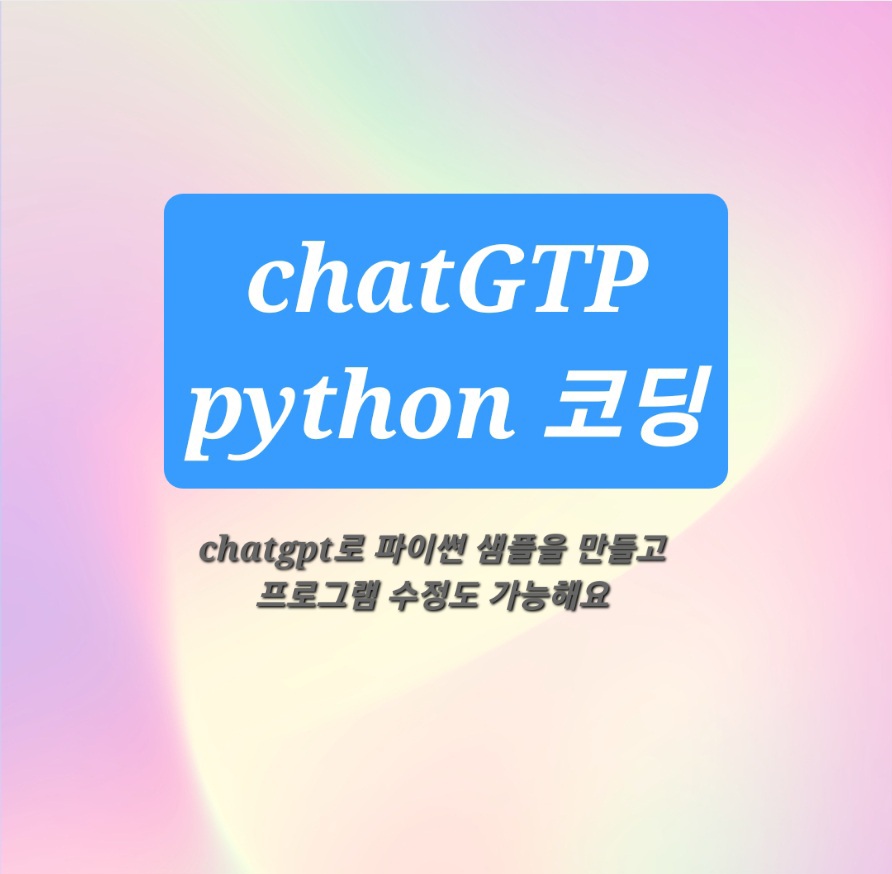
#GPT-코딩연습 #GPT로-코딩할수있다. #GPT로-배우는-파이썬코딩-연습
GPT로 파이썬 코딩을 하는데 파일을 읽고 쓰는 부분에 대한 내용을 다루어 보고자 합니다.
GPT가 좋은 것은 내가 원하는 샘플 코드를 작성을 해주므로 초급자들도 쉽게 따라할 소스들을 만들 수 있습니다.
그 만큼 샘플 코딩의 시간을 줄여 주므로 자리에 않아서 코딩하지 않아도 된다는 점이 좋습니다.
코딩하면서 궁금한 상황이 있을때 인터넷을 검색 했던 예전과 달리 아주 원하는 샘플을 GPT로 만들어 달라고하면 쉽게 볼수가 있습니다.
우선 GPT와 소통을 위해서는 코딩을 해달라는 메시지와 함께 구체적인 내용 부터 시작을 해야합니다.
[GPT에게 질문하는 방법]
chatGPT와 소통하는 방식은 사람과 이야기 하는 것과 같습니다.
1. 우선 간단한 예시를 만들어 달라고 합니다.
2. GPT의 응답은 샘플 코드와 설명을 주저리 달아 줍니다.
3. 그리고 답변 받은 내용에서 추가하고 싶은 내용을 해달라고 요청하면 됩니다.
간단하기 는 한데 말로써야 하니 어렵기도 합니다.
1. 파일을 읽고 리스트에 저장하는 샘플 작성
[질문] show me an example program of read file into list by python
[응답] Certainly! Here's an example program in Python that reads the contents of a file into a list:
# Open the file for reading
with open('example.txt', 'r') as file:
# Read the contents of the file into a list
lines = file.readlines()
# Print the list of lines
print(lines)
In this example, we're assuming that the file we want to read is called 'example.txt', and it's located in the same directory as the Python script.
The with
statement ensures that the file is properly closed after we're done reading it, even if an exception occurs. We open the file in read mode ('r'
), which allows us to read the contents of the file without modifying it.
The readlines()
method reads the contents of the file into a list of strings, with each string representing a single line of the file.
Finally, we print the contents of the list to verify that the file was read correctly.
Note that the list returned by readlines()
will include the newline character ('\n'
) at the end of each line. If you want to remove this character, you can use the strip()
method like so:
# Open the file for reading
with open('example.txt', 'r') as file:
# Read the contents of the file into a list
lines = [line.strip() for line in file.readlines()]
# Print the list of lines
print(lines)
This version of the program will remove the newline character from each line before adding it to the list.
----------
2. 추가 질문으로 import 문을 추가 해달라고 합니다.
import 문장을 구성하도록 요청을 합니다.
[ 질문 ] add some imports statements.
[응답] Sure! Here's the same program with some additional imports that might be needed depending on what you want to do with the list of lines:
import os # for checking file existence
import sys # for handling errors
# Check if the file exists
if not os.path.exists('example.txt'):
print("Error: file 'example.txt' does not exist")
sys.exit(1)
# Open the file for reading
with open('example.txt', 'r') as file:
# Read the contents of the file into a list
lines = file.readlines()
# Print the list of lines
print(lines)
The os
module is used to check if the file exists before trying to open it. If the file doesn't exist, we print an error message and exit the program using sys.exit(1)
.
Depending on what you want to do with the list of lines, you might also need to import other modules like re
(for regular expressions), numpy
(for numerical operations), or pandas
(for data analysis). However, these imports are not strictly necessary for reading a file into a list.
3. 추가 요청으로 함수문장으로 변환해 봅니다.
* 추가적인 질문으로 샘플을 함수문장으로 작성을 요청하여 보았습니다.
[질문] transform it with def function
[응답] Sure, here's an example of how you could refactor the previous code into a function:
import os
import sys
def read_file_into_list(filename):
if not os.path.exists(filename):
print(f"Error: file '{filename}' does not exist")
return None
with open(filename, 'r') as file:
lines = file.readlines()
return lines
This function takes a filename argument and returns a list of the lines in the file. If the file doesn't exist, it prints an error message and returns None.
To use this function, you would call it with the name of the file you want to read, like so:
lines = read_file_into_list('example.txt')
if lines is not None:
print(lines)
4. 리스트를 파일로 저장하는 예시
다음 추가적인 질문은 리스트를 다시 파일로 저장하는 것인데 ..이것도 개발자가 많이 필요한 내용을 작성해 주었습니다.
data = [ 'apple','banana', 'cherry' , 'date' ]
with open ('output.txt' , 'w' ) as file:
for item in data:
file.write(f"{item}\n")
이 예에서는 4개의 문자열이 포함된 데이터 목록( data)으로 시작합니다. 그런 다음 함수를 사용하여 쓰기 위해 'output.txt'라는 파일을 엽니다 open(). 인수 'w'는 Python에게 쓰기 모드로 파일을 열도록 지시합니다. 즉, 파일에 데이터를 쓸 수 있습니다.
그런 다음 루프를 사용하여 목록 for의 각 항목을 반복합니다 data. 각 항목에 대해 메서드를 사용하여 파일에 기록합니다 write(). '\n' 문장 으로 파일에서 각 항목이 별도의 줄에 있도록 각 항목 뒤에 개행 문자를 추가합니다 .
마지막으로 with 문장 블록이 종료 하면 with의 파일이 자동으로 닫힙니다. '끝'
'Programming' 카테고리의 다른 글
[Python] SQLAlchemy: 객체 기반 데이터 활용 SQLAlchemy ORM (Object Relational Mapping)(1) (0) | 2023.04.05 |
---|---|
인기있는 모바일 개발에 필요한 파이썬 개발툴 (0) | 2023.03.08 |
(python) chatGPT를 이용한 String-Buffer로 파일 쓰기 (0) | 2023.03.02 |
(자료구조) Linkedlist - 연결 리스트 구조와 종류별 python examples (0) | 2022.12.09 |
(쿨팁) git 에서 https repository 연결시 SSL 인증서 오류 해결법 http.sslVerify false (0) | 2022.11.24 |
추천-파이썬-모바일-개발툴-Python-editor-2022 (0) | 2022.11.07 |
(쿨팁)pip SSLCertVerificationError with --trusted-host (0) | 2022.08.02 |